Intro
In an era where instant and interactive customer service is the norm, Amazon Lex emerges as a transformative solution, enabling businesses to build sophisticated, AI-powered chatbots and voice assistants.
This technology leverages the same deep learning functionalities that power Amazon Alexa, making it possible to design conversational interfaces that genuinely understand the user's intent.
Amazon Lex automates interactions, offering a seamless, 24/7 customer engagement model that scales as your business grows.
This not only enhances user experience but also significantly reduces operational costs.
Business Needs
In today's fast-paced digital landscape, companies face a critical challenge: meeting customer demands for immediate support without straining their resources.
Long wait times and inadequate responses can lead to frustration and lost trust. There is a pressing need for solutions that enhance service efficiency while minimizing operational costs.
As customer expectations continue to rise, the inability to provide personalized interactions risks driving customers away.
Therefore, leveraging conversational AI like Amazon Lex becomes essential in effectively addressing these pain points.
Introducing Amazon Lex
Amazon Lex is a powerful service that enables businesses to build conversational interfaces using voice and text, effectively addressing the challenges of providing timely and accurate customer support.
By automating interactions, it significantly reduces response times and improves user satisfaction.
Key Features:
- Natural Language Understanding (NLU): Amazon Lex uses advanced machine learning to understand user intents and context, improving the accuracy of interactions.
- Multi-Channel Support: The service allows seamless integration with various platforms, including web applications, mobile apps, and messaging services, ensuring a consistent user experience.
- Scalability: Lex is designed to easily scale with your business needs, efficiently handling increasing interaction volumes as your customer base grows.
Key Concepts in Amazon Lex
To effectively utilize Amazon Lex, it is essential to understand its key concepts:
- Utterance: An utterance is what the user says or types to interact with the chatbot. It represents the user's input, which Lex will interpret to determine the desired action.
- Intent: An intent is a mapping between what the user wants to accomplish and a specific action that the application should take. Each intent corresponds to a unique user goal, such as retrieving information or performing an action.
- Slots: Slots are variables that capture specific pieces of information from the user's utterance needed to fulfill an intent. They act as placeholders that get filled in with data extracted from the user's input, such as dates or locations.
Consider the following examples of user utterances that correspond to an intent for retrieving warehouse movements:
- Get all movements for warehouse {warehouse} on {date}
- Give me all movements from warehouse {warehouse} on {date}
In these examples, {warehouse} and {date} are slots that will be populated with the specific warehouse name and date provided by the user, allowing the system to retrieve the relevant information.
A First, Simple Scenario
Imagine needing a chatbot integrated into an inventory management system. When a user interacts with the chatbot, it triggers Amazon Lex, which processes the user's request. Lex then invokes an AWS Lambda function that interprets the prompt and queries data stored in DynamoDB. After retrieving the necessary information, the Lambda function formats the response and sends it back to Lex. Finally, Lex delivers this information back to the user, ensuring a seamless and efficient interaction.
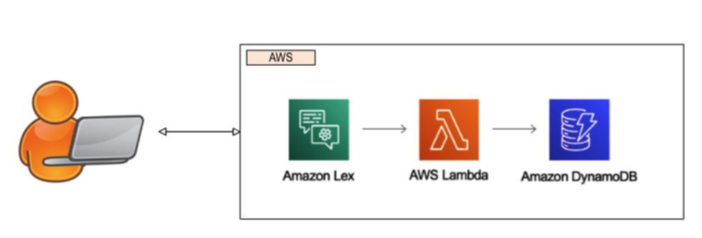
Let’s inspect the lambda
lambda_handler.py
from warehouse_service import get_movements_by_warehouse_and_date
def lambda_handler(event, context):
slots = event['currentIntent']['slots']
warehouse = slots['warehouse']
date = slots['date']
movements = get_movements_by_warehouse_and_date(warehouse, date)
if movements:
movement_list = ", ".join(m.__str__() for m in movements)
response_message = f"Sure, here is the list of movements: {movement_list}."
else:
response_message = "There are no recorded movements for the given warehouse and date."
return {
'dialogAction': {
'type': 'Close',
'fulfillmentState': 'Fulfilled',
'message': {
'contentType': 'PlainText',
'content': response_message
}
}
}
warehouse_service.py
import boto3
from boto3.dynamodb.conditions import Key
from botocore.exceptions import ClientError
from warehouse_movement_item import WarehouseMovementItem
TABLE_NAME = 'devrel-chatbot-dev-warehouse'
def get_movements_by_warehouse_and_date(warehouse: str, operation_date: str) -> list[WarehouseMovementItem]:
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table(TABLE_NAME)
try:
response = table.query(
KeyConditionExpression=Key('pk').eq(warehouse) &
Key('sk').eq(operation_date)
)
items = response.get('Items', [])
return [WarehouseMovementItem.from_dict(item) for item in items]
except ClientError as e:
print(f"Failed to retrieve items: {e.response['Error']['Message']}")
return []
This is the DynamoDB table’s data:
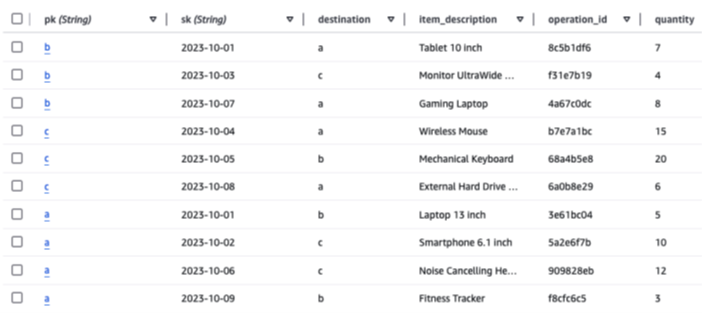
Setup the ChatBot with IaC with Terraform
Terraform is an open-source tool that enables Infrastructure as Code (IaC), allowing you to define and provision cloud resources through configuration files.
The entire infrastructure, including AWS Lambda and DynamoDB, has already been provisioned with Terraform.
Below are code snippets to set up a chatbot using Amazon Lex with Terraform.
resource "aws_lex_bot" "warehouse_bot" {
name = "WarehouseBot"
description = "Chatbot for warehouse movements queries"
process_behavior = "BUILD"
idle_session_ttl_in_seconds = 300 # 5 minutes
enable_model_improvements = true
locale = "en-US"
child_directed = false
abort_statement {
message {
content = "Sorry, I couldn't process your request. Please try again."
content_type = "PlainText"
}
}
clarification_prompt {
max_attempts = 2
message {
content = "I didn't understand you. Could you please rephrase your request?"
content_type = "PlainText"
}
}
intent {
intent_name = aws_lex_intent.warehouse_movements.name
intent_version = aws_lex_intent.warehouse_movements.version
}
}
resource "aws_lex_intent" "warehouse_movements" {
name = "GetWarehouseMovements"
description = "Intent to get warehouse movements for a specific date"
sample_utterances = [
"Get all movements for warehouse {warehouse} on {date}",
"Show me movements for warehouse {warehouse} on {date}",
"List all warehouse {warehouse} movements for {date}",
"Give me all movements from warehouse {warehouse} on {date}"
]
fulfillment_activity {
type = "CodeHook"
code_hook {
message_version = "1.0"
uri = aws_lambda_function.chatbot_lambda_function.arn
}
}
slot {
name = "warehouse"
description = "The warehouse identifier"
slot_constraint = "Required"
slot_type = "AMAZON.AlphaNumeric"
value_elicitation_prompt {
max_attempts = 2
message {
content = "Which warehouse are you interested in?"
content_type = "PlainText"
}
}
}
slot {
name = "date"
description = "The date of movements"
slot_constraint = "Required"
slot_type = "AMAZON.AlphaNumeric"
value_elicitation_prompt {
max_attempts = 2
message {
content = "For which date would you like to see movements?"
content_type = "PlainText"
}
}
}
}
resource "aws_lambda_permission" "lex_invoke_permission_generic" {
statement_id = "AllowExecutionFromLexGeneric"
action = "lambda:InvokeFunction"
function_name = aws_lambda_function.chatbot_lambda_function.function_name
principal = "lex.amazonaws.com"
}
Linking Lambda and the Chatbot
The connection between the AWS Lambda function and the chatbot in Amazon Lex is established through the “fulfillment_activity” section, utilizing a code hook. This code hook specifies that the Lambda function will be invoked to handle the logic required to fulfill the user's intent.
When a user interacts with the chatbot and their request is matched to a specific intent, the corresponding Lambda function is triggered, allowing it to process the necessary data and return a response back to Amazon Lex for the user.
This integration ensures dynamic and efficient handling of user interactions.
Let's See the Chatbot in Action
Using the AWS Management Console, it is possible to test the chatbot in real-time, allowing users to interact with it directly and observe its responses to different inputs.
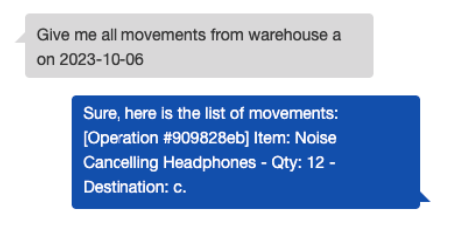
A More Complex Scenario - Leveraging Generative AI with Bedrock
Now that the foundational components for building a chatbot have been established, it's possible to explore more advanced functionalities by integrating the Lambda function not only with DynamoDB but also with powerful services like Amazon Bedrock. Amazon Bedrock is a fully managed service that makes it easier for developers to build and scale generative AI applications using foundation models.
By leveraging Bedrock, businesses can enhance user interactions by utilizing advanced natural language processing capabilities, enabling more nuanced conversations with customers. This integration can significantly improve the chatbot's ability to understand complex queries and deliver more insightful responses.
From a business perspective, this translates to a superior customer experience, as users can engage in more meaningful dialogues and obtain relevant information quickly. Moreover, by providing a richer interaction model, companies can drive higher customer satisfaction and loyalty, ultimately leading to increased operational efficiency and effectiveness in meeting user needs.
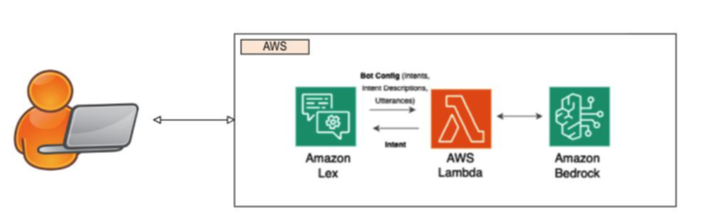
Conclusion
In an increasingly competitive landscape, businesses face the critical challenge of meeting customer expectations for fast and effective support. By addressing these needs through innovative solutions, companies can enhance customer satisfaction and operational efficiency. Generative AI serves as a powerful tool in this regard, enabling more sophisticated interactions that go beyond basic queries.
Integrating a chatbot with advanced services, such as Amazon Bedrock, empowers businesses to create dynamic and meaningful conversations with users. These interactions not only provide immediate answers but also foster a deeper understanding of customer needs.
AWS stands out as a leader in offering a comprehensive suite of interconnected services that can be easily assembled to meet varying business demands. By leveraging AWS's powerful tools, organizations can build robust solutions that align with both user expectations and business objectives, ultimately driving growth and success in their respective markets.
For a practical example of these concepts in action, you can explore the repository at this GitHub link.